Accept Crypto Payments with Coinbase Commerce & OnchainKit
Accepting crypto payments can help you eliminate traditional credit card fees and avoid costly chargebacks, giving you a faster, more global payment experience. In this guide, you'll learn how to quickly integrate Coinbase Commerce and OnchainKit to accept crypto payments for products or services in your application.
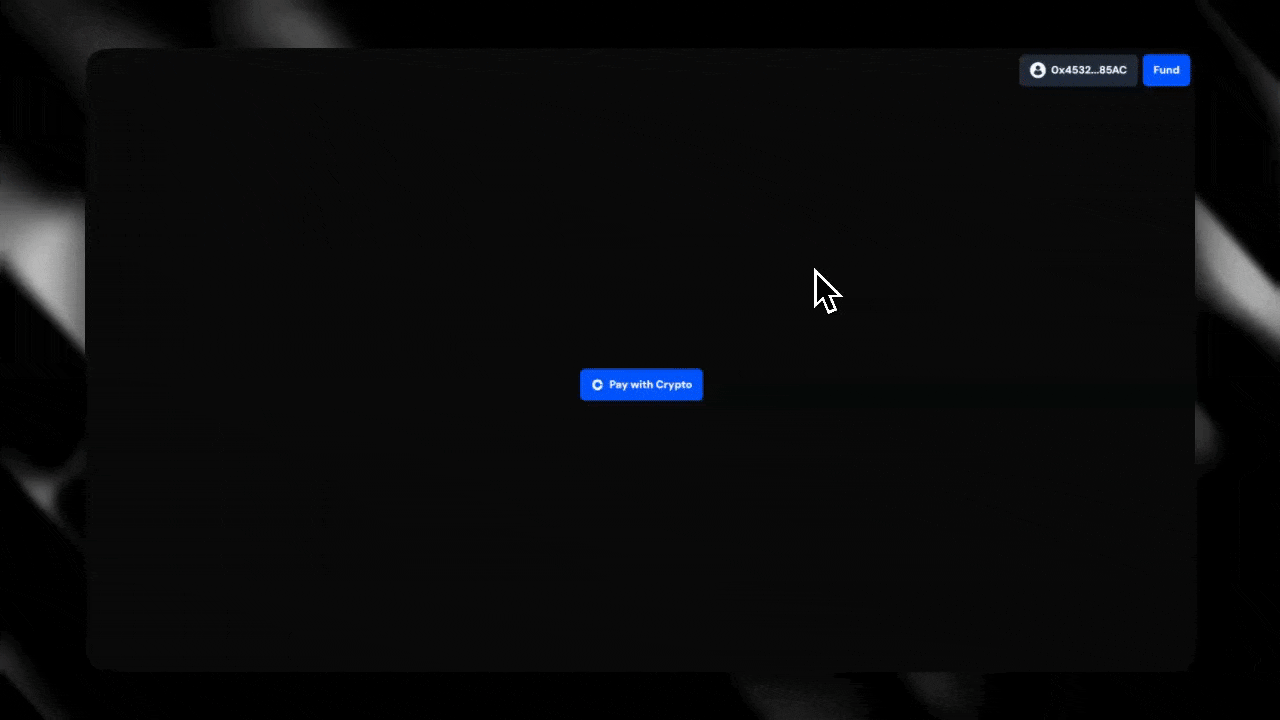
Objectives
By following this guide, you will learn how to:
- Create or configure a product in Coinbase Commerce
- Configure your OnchainKit environment
- Implement a checkout flow for accepting crypto payments
- Deploy and test your app to confirm the payment flow
Prerequisites
1. Coinbase Commerce Account
Coinbase Commerce allows you to accept cryptocurrency payments globally. Sign up to get started.
2. Coinbase Developer Platform (CDP) Account
Coinbase Developer Platform (CDP) provides the tools and APIs you need for integration.
Step-by-Step Setup
Step 1: Create a Product in Coinbase Commerce
- Log in to your Coinbase Commerce account.
- Go to the product creation page.
- Add product details (name, description, price).
- Click Create product.
- Once created, select View product and copy the UUID from the URL.
Tip: Store the product UUID as an environment variable in your .env
file. This makes it easier to reference safely in your code.
Step 2: Clone the OnchainKit App Template
Use the official OnchainKit app template to bootstrap your project:
npm create onchain@latest
Step 3: Configure Environment Variables
In the project folder, open (or create) your .env
file and add:
NEXT_PUBLIC_ONCHAINKIT_PROJECT_NAME=
NEXT_PUBLIC_ONCHAINKIT_API_KEY=
NEXT_PUBLIC_PRODUCT_ID=
Note:
NEXT_PUBLIC_PRODUCT_ID
should be set to the UUID from your Coinbase Commerce product.NEXT_PUBLIC_ONCHAINKIT_API_KEY
should be your CDP API key.
Step 4: Implement the Payment Component
-
Open
app/page.tsx
(or similar entry page). -
Import the necessary components:
import { Checkout, CheckoutButton, CheckoutStatus } from '@coinbase/onchainkit/checkout'; import Image from 'next/image'; const productId = process.env.NEXT_PUBLIC_PRODUCT_ID;
-
Create a folder to house the image of your product or service in
app/public
-
Add an image of your product or service in the
public
folder (e.g.,app/public/my-pizza.jpeg
). -
Display that image and conditionally render the checkout UI only when a wallet is connected:
app/page.tsx<section className='flex w-full flex-col items-center justify-center gap-8 px-2 py-4'> <h1 className='pixel-font text-xl md:text-2xl mb-8 text-center'> ONCHAIN PIZZA </h1> <div className='w-[300px] h-[300px] relative'> <Image src='/8bitpizza.png' fill style={{ objectFit: 'contain' }} alt='8-bit pizza' className='rounded-xl' priority /> </div> <div className='mt-6'> {address ? ( <Checkout productId={productId}> <CheckoutButton coinbaseBranded={true} /> <CheckoutStatus /> </Checkout> ) : ( <Wallet> <ConnectWallet> <Avatar className='h-6 w-6' /> <Name /> </ConnectWallet> </Wallet> )} </div> </section>
Step 6: Test & Deploy
- Run the development server:
- Visit
http://localhost:3000
to confirm that the payment button works for your product or service. - Deploy with your preferred hosting provider (e.g., Vercel).
Conclusion
Congratulations! You've successfully integrated Coinbase Commerce and OnchainKit into your application, enabling crypto payments. By providing this option, you can:
- Eliminate traditional payment fees and chargebacks
- Expand your audience to crypto users worldwide
- Easily scale your offerings for more products and services
Happy building and enjoy the benefits of a global, decentralized payment system on Base!